mirror of
https://github.com/revanced/revanced-patcher.git
synced 2025-05-09 16:44:25 +02:00
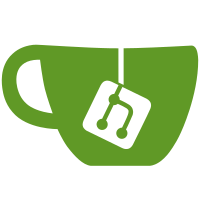
Old methods have been marked as deprecated, and will be removed in the future. - ResourceData.kt was made an Iterable<File>, and the forEach method was removed in favor of Kotlin's forEach function. (no modifications required) - The resolve method was deprecated in favor of a new operator getter function, which can be either called using get(path) or data[path]. This keeps backwards compatibility with the old get method. - The getXmlEditor method was deprecated in favor of the new xmlEditor variable, which is a XmlFileHolder which has an operator getter which acts like an array. This is syntactically better.
35 lines
1.4 KiB
Kotlin
35 lines
1.4 KiB
Kotlin
package app.revanced.patcher.data.impl
|
|
|
|
import app.revanced.patcher.data.Data
|
|
import org.w3c.dom.Document
|
|
import java.io.Closeable
|
|
import java.io.File
|
|
import javax.xml.parsers.DocumentBuilderFactory
|
|
import javax.xml.transform.TransformerFactory
|
|
import javax.xml.transform.dom.DOMSource
|
|
import javax.xml.transform.stream.StreamResult
|
|
|
|
class ResourceData(private val resourceCacheDirectory: File) : Data, Iterable<File> {
|
|
operator fun get(path: String) = resourceCacheDirectory.resolve(path)
|
|
val xmlEditor = XmlFileHolder()
|
|
override fun iterator() = resourceCacheDirectory.walkTopDown().iterator()
|
|
|
|
inner class XmlFileHolder {
|
|
operator fun get(path: String) = DomFileEditor(this@ResourceData[path])
|
|
}
|
|
|
|
@Deprecated("Use operator getter instead of resolve function", ReplaceWith("get(path)"))
|
|
fun resolve(path: String) = get(path)
|
|
|
|
@Deprecated("Use operator getter on xmlEditor instead of getXmlEditor function", ReplaceWith("xmlEditor[path]"))
|
|
fun getXmlEditor(path: String) = xmlEditor[path]
|
|
}
|
|
|
|
class DomFileEditor internal constructor(private val domFile: File) : Closeable {
|
|
val file: Document = DocumentBuilderFactory.newInstance().newDocumentBuilder()
|
|
.parse(domFile).also(Document::normalize)
|
|
|
|
override fun close() = TransformerFactory.newInstance().newTransformer()
|
|
.transform(DOMSource(file), StreamResult(domFile.outputStream()))
|
|
}
|